Functional Programming: Is It The Future of Software Development?
Written on
Chapter 1: The Rise of Functional Programming
In the ever-evolving realm of programming, various paradigms have emerged and faded. The pressing question is: Does Functional Programming hold a permanent place in this landscape, or is it simply another passing trend?
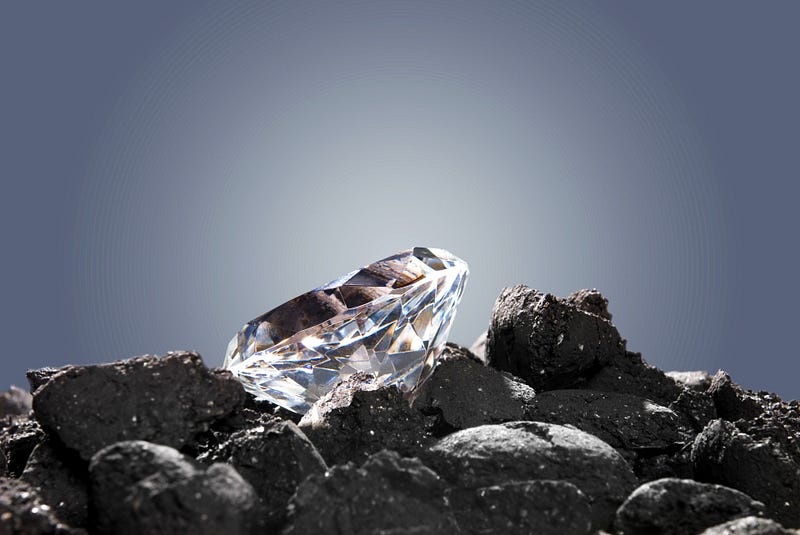
Programming languages, frameworks, and paradigms like Object Oriented Programming serve as tools for developers. Recently, Functional Programming has surged in popularity. But how does it fit into the larger picture? Is it just another tool, or could it be a transformative solution to our programming challenges?
Let’s dive deeper! What do we mean by a "silver bullet"?
A silver bullet is a means to solve all problems efficiently. Could mathematics be considered such a silver bullet? It’s certainly close!
We owe this invaluable tool to countless brilliant minds throughout history, including Euclid, Pythagoras, Archimedes, and many more. Mathematics offers predictability; for instance, 2 + 2 will always equal 4. This reliability is crucial for the advancement of science and technology.
Imagine a world where basic operations yield inconsistent results. We’d likely still be stuck in a primitive state. In medicine, for example, the lack of rigorous trials in the past led to many ineffective and dangerous practices.
Shouldn’t software also strive for predictability? Absolutely! Yet today’s software landscape can often feel chaotic, reminiscent of early medicine. Even straightforward operations, like adding two numbers, can yield incorrect results or crash entirely.
What causes these inconsistencies? What hinders software reliability?
Predictability
To ensure software reliability, it must be predictable, much like simple mathematical functions. So, how can we achieve predictability in software development?
The answer lies in avoiding nondeterminism. In computer science, a nondeterministic algorithm can produce different outcomes even with identical inputs, contrasting with deterministic algorithms.
Consider a simple function call. If it consistently returns the same output for the same input, it's deterministic. In contrast, another function may yield varying results, indicating its nondeterministic nature.
What differentiates the two? A function that relies solely on its input and does not depend on external factors is deterministic. Conversely, a function that interacts with external states is nondeterministic.
Deterministic code is inherently predictable, while nondeterministic code is fraught with unpredictability.
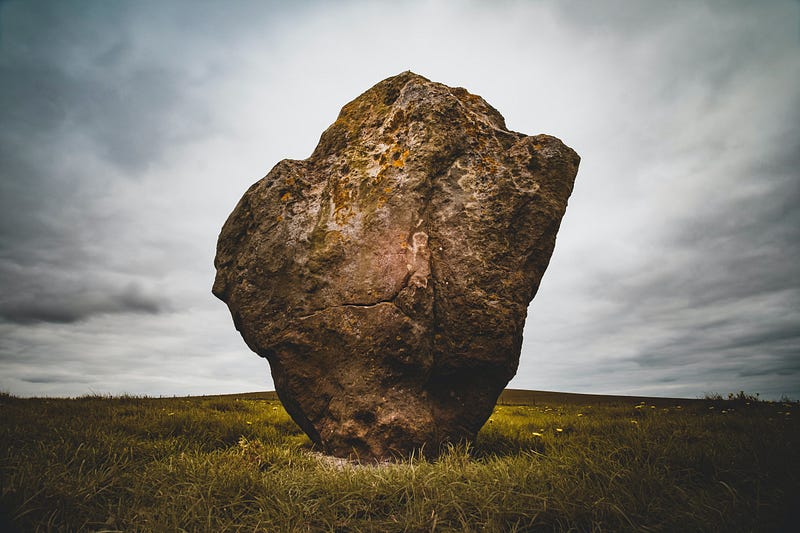
Consider the addition function: when we input (2, 2), the output will always be 4. This reliability stems from the hardware implementation of addition in most programming languages.
Now, if we introduce side effects that modify an external value, the outcome becomes unpredictable.
In summary, deterministic programs guarantee that 2 + 2 equals 4, while nondeterministic programs may occasionally yield unpredictable results, leading to frustrating bugs.
The implications of nondeterministic code are significant: software defects can result in time-consuming debugging and diminished user experiences.
To enhance reliability, we must first tackle the issue of nondeterminism.
Is Functional Programming Deterministic?
Functional Programming emphasizes pure functions, which are deterministic by nature. Other paradigms may not prioritize function purity, making it impossible to assure deterministic behavior.
In essence, pure Functional Programming stands as the only paradigm that guarantees predictability.
Avoiding Mutable State
How can we ensure our code remains deterministic? By steering clear of mutable state.
Mutable state often complicates debugging and understanding program flow, reminiscent of the difficulties posed by the once-common goto statement.
Consider this example:
Line 11: Before: [3, 5, 4, 1, 2, 9]
Line 15: After: []
Surprised? This showcases mutable state at work, causing elements to vanish unexpectedly.
To maintain discipline in state management, many developers turn to state-management libraries, yet improper usage can diminish their effectiveness.
Embracing Immutable State
Immutable state is a straightforward concept: functions should return new values without altering the originals. This practice is gaining traction, particularly in modern UI libraries like React.
Immutable state means that, unlike mutable data, the original data remains unchanged. For instance, capitalizing a string generates a new string rather than modifying the existing one.
With immutable state, we eliminate concerns about thread safety, as nothing is shared or modified. This leads to cleaner, more manageable code, simplifying testing and reasoning.
Here’s an example of how to work with immutable state effectively:
const sortedArray = originalArray.sort(); // This returns a new array
Using immutable values, we can ensure our programs are easier to reason about, share information safely across threads, and maintain predictable states.
Advantages of Immutable State:
- Easier to reason about.
- Great for concurrency.
- Simplified operation chaining.
- Predictable states.
- Quick comparisons.
- Easier to test.
Disadvantages of Immutable State:
While creating new copies with each change can seem inefficient, functional programming languages utilize persistent data structures to mitigate this issue.
Persistent Data Structures
Consider how a persistent binary tree operates:
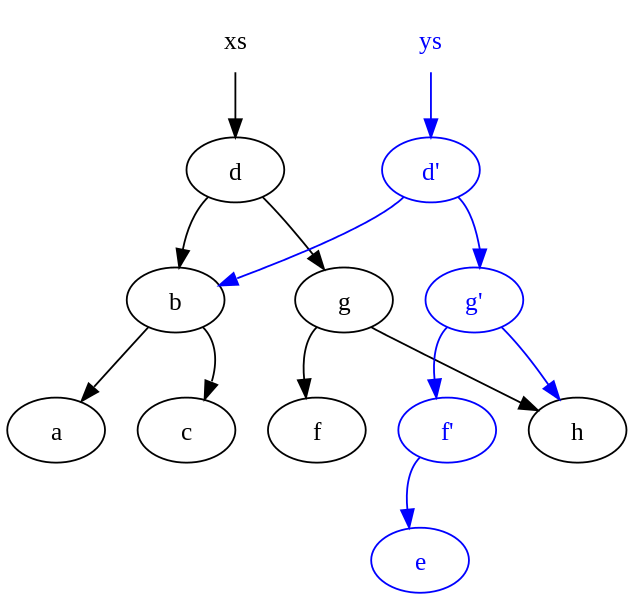
When altering a tree, the original remains intact, with new references to modified nodes. Unfortunately, mainstream languages often lack built-in support for persistent structures, making libraries like Redux and Immutable.js necessary but not ideal.
Concurrency
In the era of multi-core CPUs, effective concurrency is vital. However, many existing programming languages were designed for single-core systems, lacking adequate support for concurrent programming.
Functional Programming’s foundation in immutable state positions it perfectly for concurrent applications.
Avoiding Nulls: The Billion-Dollar Mistake
Tony Hoare, who introduced null references, referred to them as his "billion-dollar mistake." Nulls disrupt type systems, leading to runtime errors and complicating code management.
Functional programming offers alternatives like the Option pattern, which requires explicit checks for potentially absent values, enhancing safety and clarity.
Error Handling
Catching exceptions is not a sustainable error-handling strategy. While exceptions are suitable for exceptional circumstances, their frequent use can lead to unpredictable application behavior.
Instead, functional programming encourages using the Result pattern, which facilitates compile-time type checking for errors.
Programming with Pure Functions
Pure functions consistently return the same output for the same input, making them easier to test and reason about. They should not alter any state or interact with external systems.
The benefits of pure functions include:
- Simplicity in testing.
- Easier reasoning.
- Composability.
- Concurrency advantages.
Algebraic Data Types and Pattern Matching
Algebraic Data Types (ADTs) allow for powerful modeling of application state, akin to enhanced enums. Pattern matching simplifies code expression and ensures exhaustiveness checks at compile time.
Is Functional Programming a Silver Bullet?
To revisit the initial question: Is Functional Programming the ultimate solution? While not a flawless fix for all issues, it offers substantial advantages due to its mathematical foundation, particularly in lambda calculus.
By focusing on pure functions and immutability, Functional Programming enhances predictability and reliability, ultimately making software development more efficient.
In conclusion, Functional Programming is very close to being a silver bullet for the challenges faced by developers today.
What’s Next?
For a lighthearted comparison of Functional Programming and Object Oriented Programming, check out the article "Functional Programming? Don’t Even Bother, It’s a Silly Toy." Also, for insights into modern programming languages, see "These Modern Programming Languages Will Make You Suffer."
Explore the differences between Object Oriented Programming and Functional Programming in this insightful video.
Learn about the fundamental concepts of Functional Programming in an easy-to-understand format with this video.