Understanding JavaScript Promises: A Comprehensive Guide
Written on
Chapter 1: Introduction to Promises
Promises play a crucial role in JavaScript for managing asynchronous operations. They serve as a representation for tasks that are yet to be completed, hence the term "promise."
By utilizing promises, developers can effectively manage multiple asynchronous tasks, significantly minimizing the risk of "callback hell," which often results in convoluted and difficult-to-maintain code.
Why opt for promises? Here are a few compelling reasons:
- They provide a more efficient way to manage asynchronous tasks.
- They enhance error handling capabilities.
- They contribute to cleaner, more readable code.
Let’s delve deeper into how promises function.
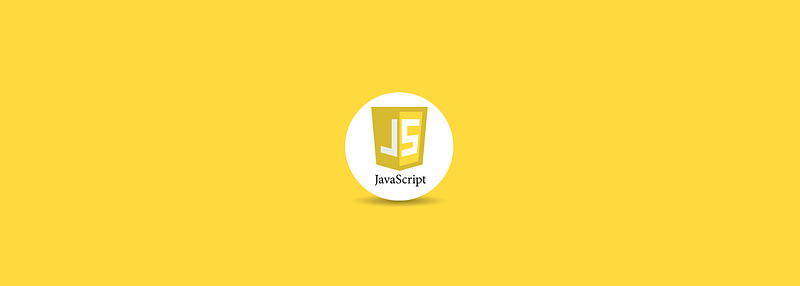
If you're eager to keep up with the latest in web development and receive updates directly in your inbox, consider subscribing to my newsletter! Join a vibrant community of designers and developers.
Chapter 2: The Mechanics of Promises
A promise can be envisioned as a placeholder for an anticipated value that is awaited upon the promise's creation. This mechanism allows developers to link event handlers to the success or failure of asynchronous actions.
In essence, asynchronous methods can return values similarly to synchronous methods. Instead of yielding an immediate result, they return a promise that will eventually provide the value.
Promises can exist in one of the following states:
- Pending: The initial state where the operation is neither fulfilled nor rejected.
- Fulfilled: The state when the operation completes successfully.
- Rejected: The state indicating the operation has failed.
- Settled: The state where the operation has either fulfilled or rejected.
Let’s examine how to create a promise:
let completed = true;
let promise = new Promise(function(resolve, reject) {
// perform an action
if (completed) {
resolve("It worked!");} else {
reject(Error("It didn't work.."));}
});
Here, the promise constructor accepts a single argument—a callback function with two parameters: resolve and reject. Within the callback, you execute the desired operation (like an asynchronous action). If successful, you call resolve; if it fails, you call reject.
It's worth noting that in this example, we reject using an Error object, which isn't necessary but can be helpful for certain debugging tools that track the stack trace.
Chapter 3: Utilizing Promises
Now, how can we utilize the promise we created?
promise.then(function(result) {
console.log(result); // "It worked!"
}, function(err) {
console.log(err); // Error: "It didn't work.."
});
The then() method accepts two arguments: one for handling success and another for dealing with failure. Both are optional, allowing you to specify a callback for either scenario as needed.
JavaScript Promises In 10 Minutes - YouTube
This video provides a succinct overview of JavaScript promises, explaining their significance and how they can enhance your programming experience.
JavaScript Promises -- Tutorial for Beginners - YouTube
In this tutorial, beginners will learn the fundamentals of promises in JavaScript and how to implement them in various scenarios.
Chapter 4: Conclusion
To summarize, a promise is an object that signifies a value that will be available in the future. It starts in the pending state and transitions to either fulfilled or rejected before being considered settled.
I hope this introductory guide has been insightful! If you have any questions, feel free to leave them in the comments below; I'm here to help!
About Me
Hello! I'm Tim, a freelance business owner, web developer, and author. I specialize in teaching both novice and experienced freelancers how to establish and sustain a successful freelancing career. For more of my articles, check out my blog.
Thank you for reading!