Mastering Laravel Queues: A Comprehensive Guide to Workflows
Written on
Introduction to Laravel Queues
Before diving into advanced topics, it's essential to grasp the basics of Laravel and its queue system.
Workflows Overview
In Laravel, rather than adding individual jobs to the queue, you can group them logically. There are two primary types of workflows: chains and batches.
Chains execute jobs sequentially. They can be described as an array of jobs that run in succession.
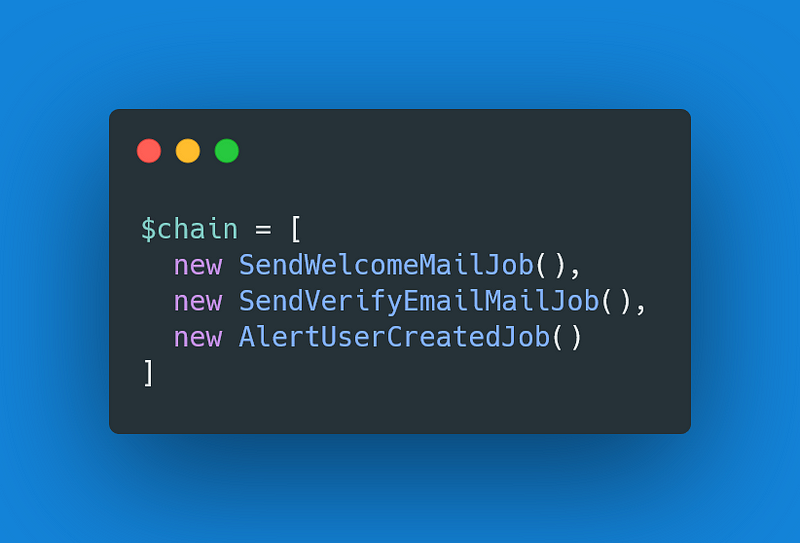
Understanding Job Chains
If any job in a chain encounters an error, the entire sequence halts. For instance, the SendVerifyEmailMailJob will not commence until the SendWelcomeMailJob has successfully completed. This rule applies to all jobs in the chain.
You can dispatch a chain to the queue using the IlluminateSupportFacadesBus.
Batches: Parallel Processing
Batches allow jobs to run concurrently without dependency on one another. The process of defining and dispatching batches is quite similar to chains.
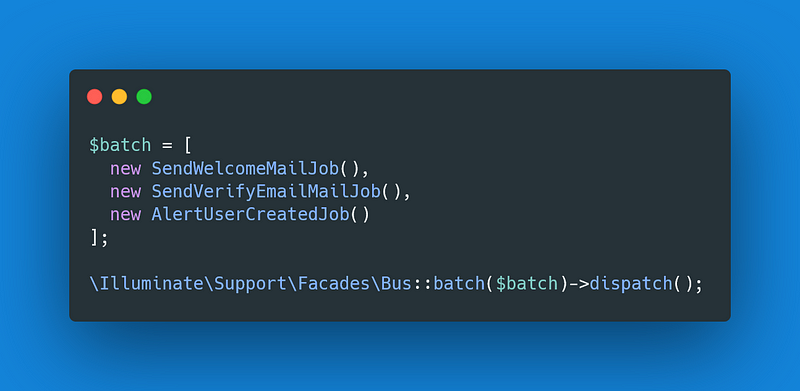
When dispatched, batches are handled by multiple workers in parallel. However, if you're utilizing the database driver, you must create a migration for your batches.
To set up your migration, execute the following commands:
php artisan queue:batches-table // Generates the migration for the batch table php artisan migrate:fresh // Migrates all tables
It's important to note that even though jobs in a batch run simultaneously, if one fails, the entire batch will be canceled. To prevent issues where one job fails while another is still attempting to run, add a check in the handle method.
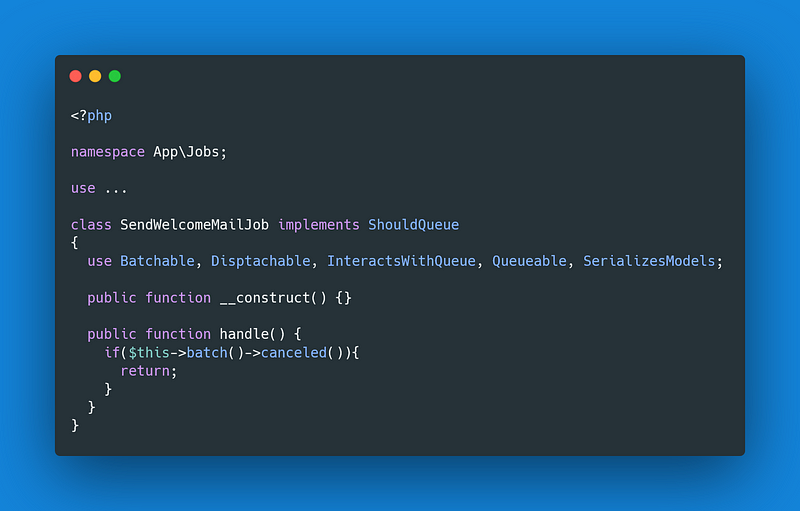
While this approach may seem straightforward, you might prefer that a single job's failure does not affect the others. To achieve this, you can invoke the allowFailures() method when dispatching the batch:
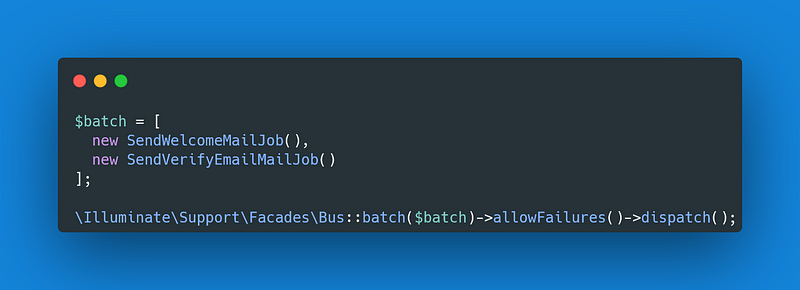
Next Steps
For a deeper understanding of advanced concepts in Laravel queues, continue to our next article:
Laravel Queue — Dispatching Workflows Advanced
This video provides a detailed overview of using queues effectively in Laravel.
In this lesson, learn about the fundamentals of queues and workers, along with how to manipulate the queue effectively.