Enhancing JavaScript Conditional Logic: Beyond If Statements
Written on
Chapter 1: Rethinking Control Flow
While if statements are a common tool for managing control flow in programming, they aren't strictly necessary. In fact, it's possible to craft extensive JavaScript code without ever using an if statement. There are numerous instances where alternative constructs can convey your intentions more clearly, which is crucial when writing code intended for human comprehension. Moreover, these alternatives often lead to less verbose and more streamlined code.
Section 1.1: Utilizing the AND (&&) Operator
The AND (&&) operator is a powerful feature in JavaScript that can simplify your code significantly. For instance, rather than writing extensive nested if statements, you can condense your logic into a single line.
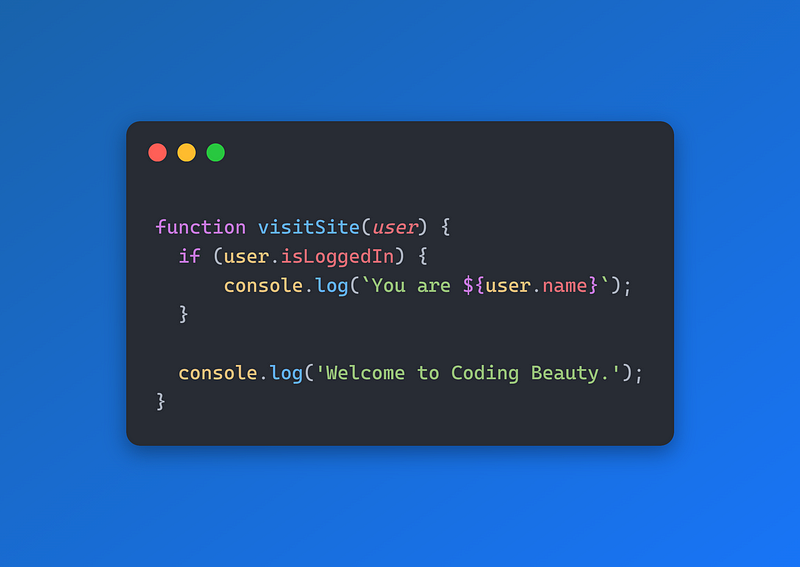
By employing the AND operator, you can convert a traditional if statement into a more concise form, especially when there's no corresponding else statement. This approach is particularly effective when your if block consists of a single line.
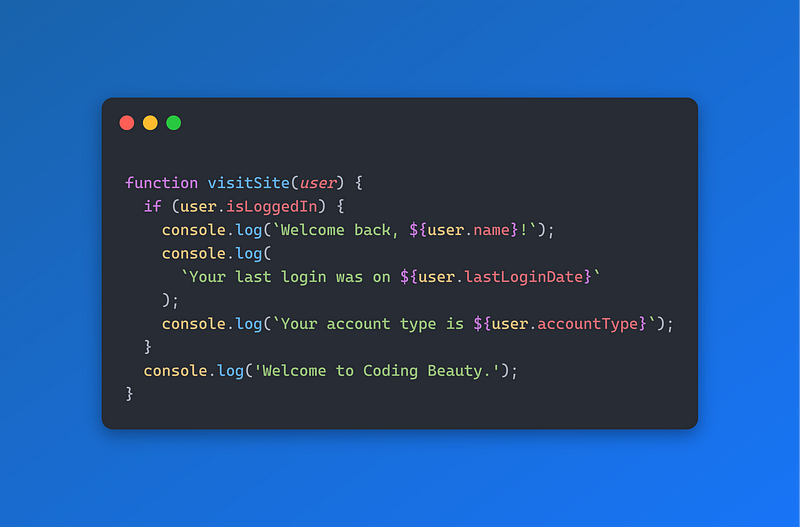
Section 1.2: The Ternary Operator
Ternary operators are another excellent way to streamline your if-else statements into a compact format. They are especially useful when you're assigning values to the same variable based on a condition.
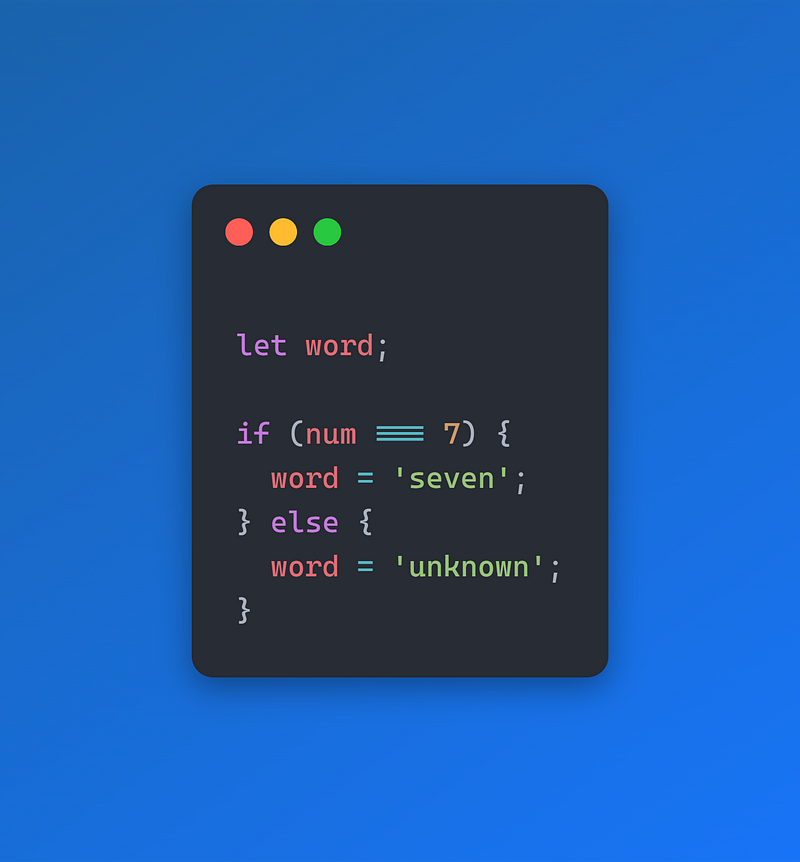
Using ternary operators can help maintain the DRY principle (Don't Repeat Yourself), resulting in cleaner code by avoiding redundant variable assignments.
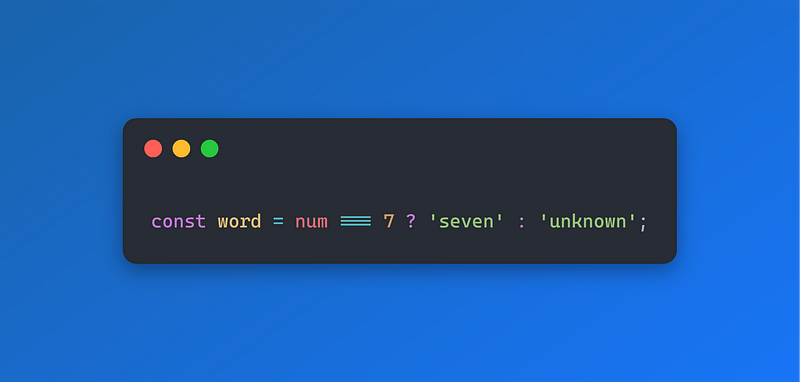
Nested ternaries can also be utilized for more complex branching scenarios, although some developers find them challenging to read. Proper formatting can greatly enhance the readability of nested ternaries, allowing you to maintain clarity even in more elaborate conditions.
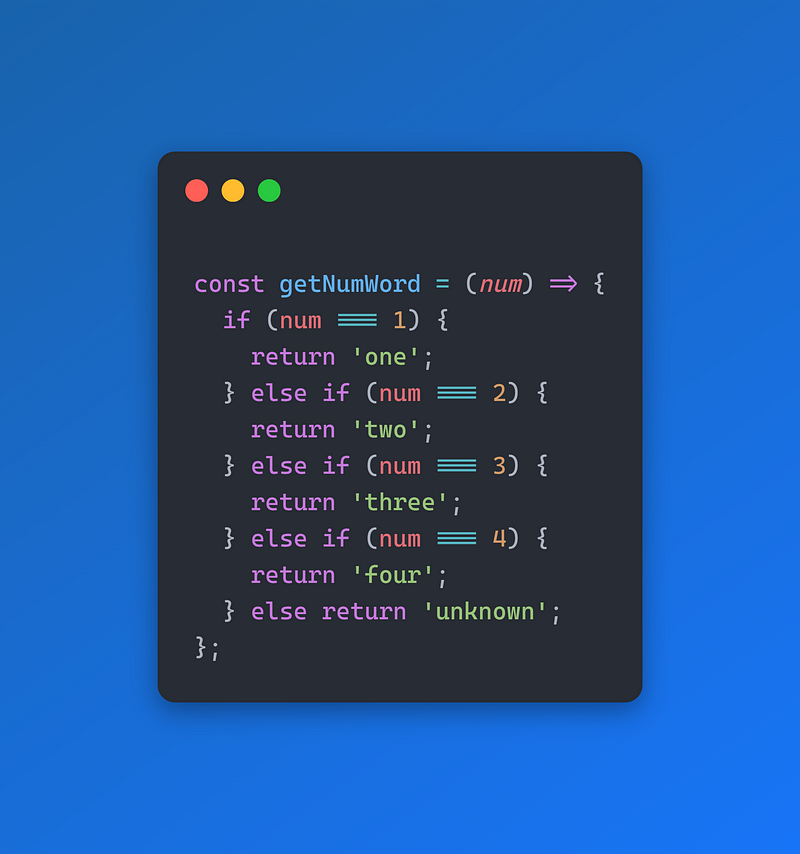
Chapter 2: Exploring Alternative Constructs
The first video titled "Nesting 'If Statements' Is Bad. Do This Instead." discusses how to avoid complex nested if statements and presents better alternatives for cleaner code.
The second video, "Why I Don't Use Else When Programming," explores the rationale behind opting out of else statements in favor of clearer programming practices.
Section 2.1: The Switch Statement
In languages like Java and C#, the switch statement serves as a powerful tool for processing input based on a single variable. Unlike traditional if statements, switch cases allow for a "fall-through" mechanism, which can simplify certain types of logic.
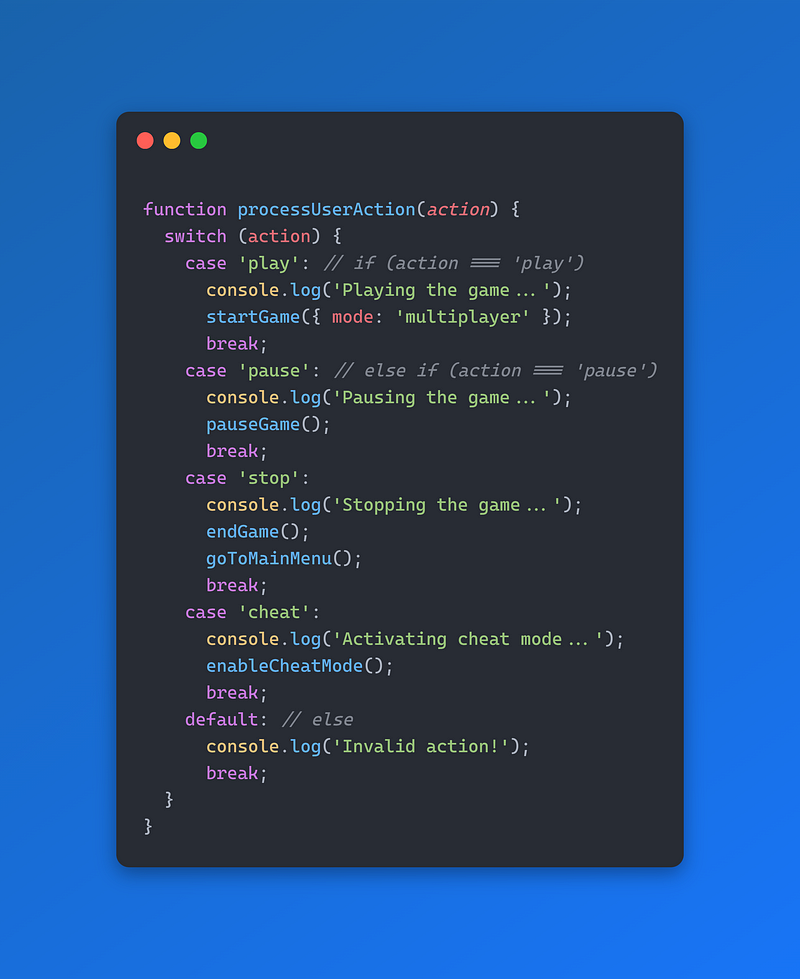
Section 2.2: Key-Value Objects
Key-value objects provide a declarative way to link inputs to outputs, making them particularly useful in frameworks like React Native where you need to handle various states (loading, error, success).
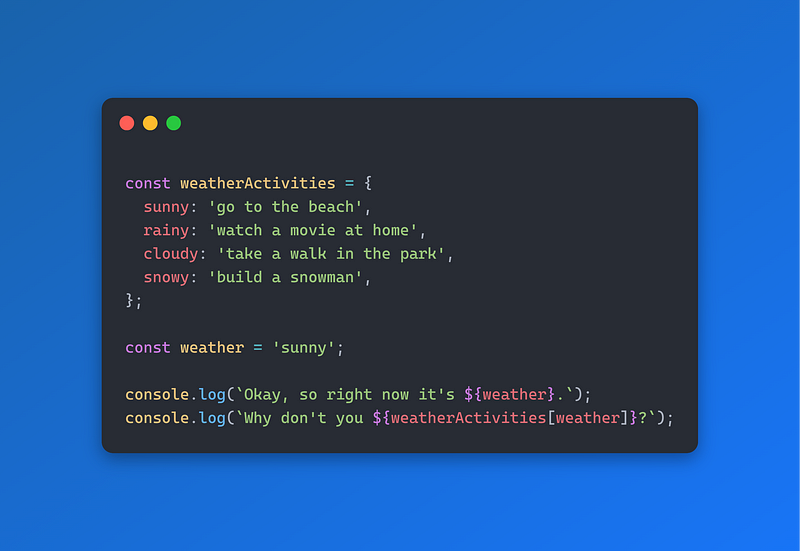
Final Thoughts
While if statements are indeed effective for crafting conditional logic, it's valuable to explore alternative methods that can lead to clearer, more efficient code. The goal isn't to eliminate if statements completely, but to adopt techniques that enhance code clarity and conciseness.
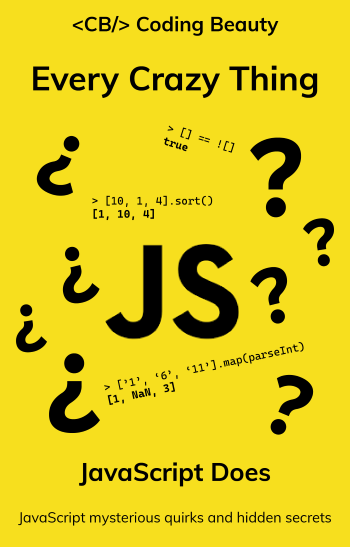